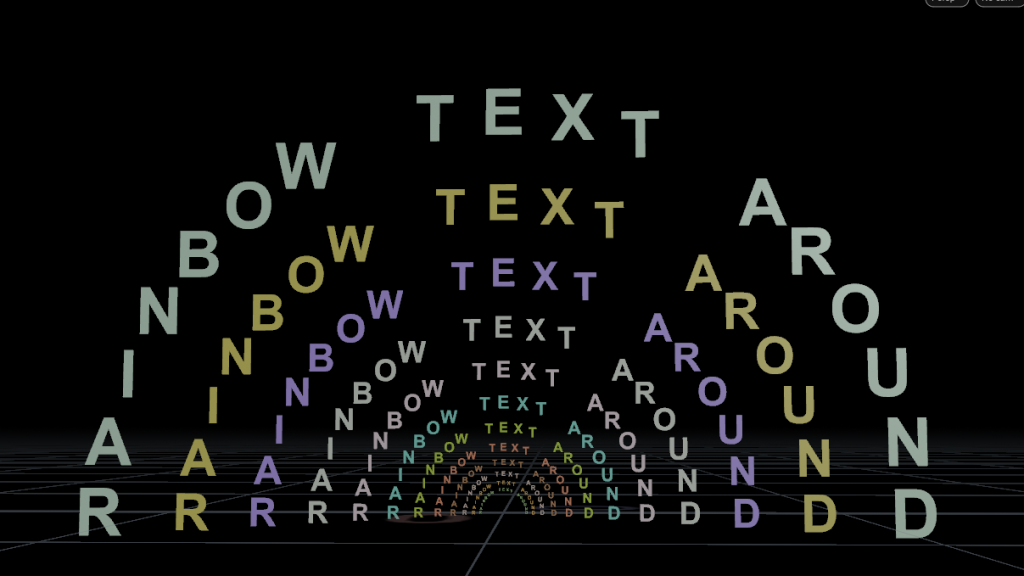
hip file: https://drive.google.com/file/d/1xQzAISBo0Bm45CLUXzmeSbKQq9OzkyJt/view?usp=drive_link
Let’s make some thread swirling around and make a net.
Firstly, we create a grid and keep only points.
We then use VOP to generate v (velocity) that we can later use it in POP to drive particle.
Here’s the note that i would like to share with you to make spherical waves by Houdini using vex to create a sphere from a grid and project wavy lines on to the surface.
Concept:
Make some wavy lines on a 2D Plane 🠒 Project them onto a Sphere (which is made from the 2D Plance) 🠒 Create Stripes based on the generated waves.
Make waves
float overall_speed = ch("overall_speed");
float leading_wave_speed = ch("leading_wave_speed");
@P.x += @Time * overall_speed;
if (@ptnum % 3 == 2)
{
@P.x += @Time * leading_wave_speed;
}
@P.x = clamp(@P.x,-5.0,5.0);
vector project_dir = chv("project_dir");
vector project_points;
vector uvw;
int primid = intersect(1, @P, project_dir, project_points, uvw);
if (primid >= 0)
{
@P = project_points;
@N = prim(1, "N", primid);
}
v@project_prim = primid;
v@project_uv = uvw;
vector relbox = relbbox(0,v@P);
float u = relbox.z;
float v = relbox.x;
float rad = ch("radius");
u *= (2*$PI);
v *= $PI;
float x = rad * sin(v) * cos(u);
float y = rad * sin(v) * sin(u);
float z = rad * cos(v);
v@P = set(x,y,z);
v@P = primuv(1, "P", i@project_prim, v@project_uv);
grid [0] – sphere [1]
apply uv for grid while sphere should be primitive or mesh (doesn’t work with polygon option)
use swizzle to re-arrange the relative bouncing box of the grid from XYZ to XZY.
use primuv to interpolate position of sphere based on attribute “P” and apply to the grid
vector toXZY = swizzle(relbbox(0,v@P),0,2,1);
vector prim_uv = primuv(1,”P”,0, toXZY);
v@P = prim_uv;
Distance from Geometry (geometry node)
Function: Measure distance between each point and a reference geometry
Example:
float max_dist = detail(1, 'max_dist');
float min_dist = detail(2, 'min_dist');
@dist = fit(@dist, min_dist, max_dist, chf('min_dist'), chf('max_dist'));
float dist_ramp = chramp('dist_ramp', @dist);
@dist *= dist_ramp;'
S2RS is a Cinema 4D plugin which loads all the textures exported from Substance Painter and create Redshift Material according to the user’s defined mapping structure.
What’s new in v1.0.2?
User Interface:
S2RS Workflow Example:
Create Cinema 4D scene and export its objects to FBX file to use in Substance Painter
From Substance Painter, set the export config to PBR MetaRough and export to a folder that you will import to Cinema 4D later.
In Cinema 4D, select the Texture Folder to load the textures you export from Substance Painter. Customize your setting and click Proceed. For applying the materials to scene, your C4D filename must be the same as your original C4D file that you export objects to Substance Painter.
//float f[] = float[](array(1, 2, s, t, length(P-L), length(N))); float array[] = float[](array(0,1,2,3,4)); int j; float temp; for (int i = 0; i < len(array); i++) { j = floor(fit01(random(i + @Time*10),0,5)); i@jv = j; temp = array[j]; } @a = temp;
Modulus operator returns the r value (remainder) after dividing a with b
r = a % b
for example:
In this example, we got a polygon circle and we choose only points with odd numbers and push them out to turn a circle into a star shape.
if (@ptnum%2==1) {
@P += @P*ch("amount");
}
To cycle the value in Carve node, we can use:
experession in First U: fit(@Time%4,1,4,0,1)
experssion in Second U: fit(@Time%4,0.1,3.5,0,1)
Add a foreach loop to create the offset for carving.
//rotate around N random
float angle = radians(chf("angle"));
float cone_angle = radians(chf("cone_angle"));
float seed = chf("seed");
vector axis = v@N;
vector rand_offset = normalize(sample_sphere_cone(axis, cone_angle, vector(rand(@ptnum + seed))));
axis = normalize(axis + rand_offset);
vector side = cross(axis, {0,1,0});
vector up = cross(axis, side);
vector front = axis;
matrix3 m = set(side, up, front);
vector4 q = quaternion(angle, axis);
vector4 orient = quaternion(m);
p@orient = qmultiply(q, orient);
float angle = radians(chf("angle"));
vector axis = v@N;
vector side = cross(axis, {0,1,0});
vector up = cross(axis, side);
vector front = axis;
matrix3 m = set(side, up, front);
vector4 q = quaternion(angle, axis);
vector4 orient = quaternion(m);
p@orient = qmultiply(q, orient);